Asking for a Tip on the Card Reader
Your merchants can easily ask their guest for a tip using On-Reader Tipping. At the start of each transaction, the guest can be asked if they want to give a tip and then can enter the tip amount directly on the card reader. You can also ask the guest to enter the total amount for the transaction, including a potential tip.
On-reader tipping is currently only available on the Miura card readers. To activate this feature, contact your account manager.
Asking for the Tip Amount
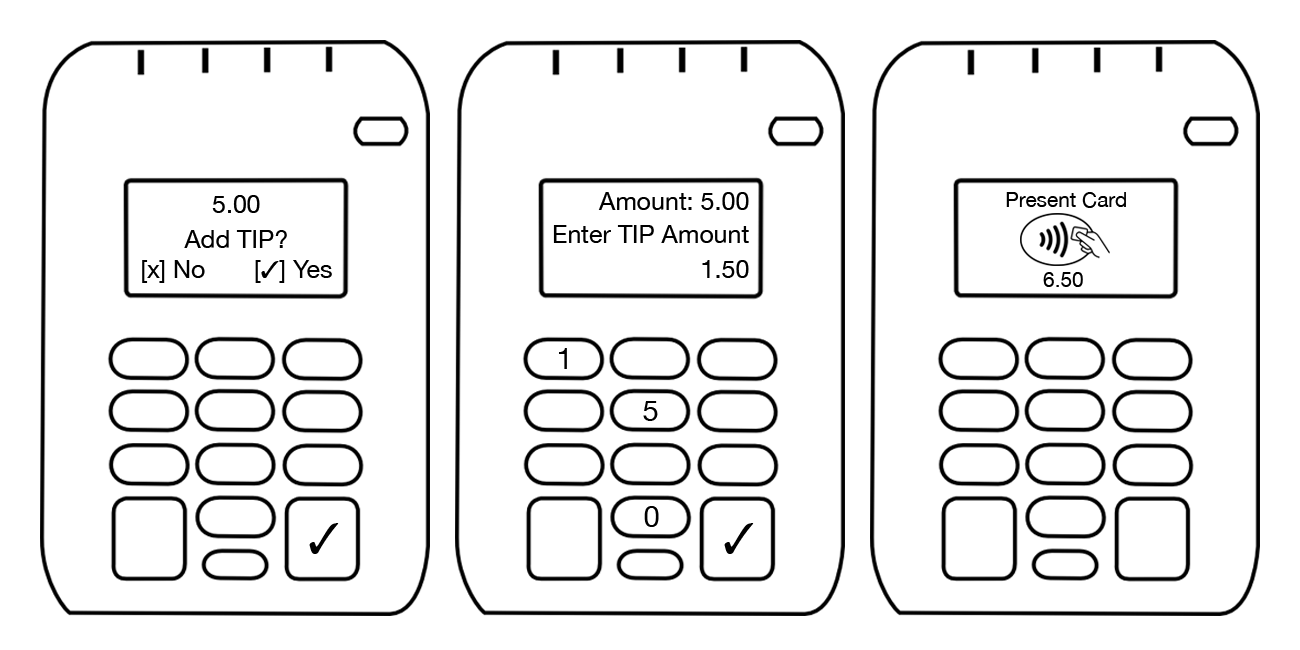
In order to ask the shopper to enter the tip amount, create
MPTransactionProcessTippingStepParameters
with tippingAmountParametersWithOptionalsBlock
and use them to create the MPTransactionProcessParameters
. Then pass them along as processParameters
to createTransactionViewControllerWithTransactionParameters
:MPTransactionProcessTippingStepParameters *params = [MPTransactionProcessTippingStepParameters tippingAmountParametersWithOptionalsBlock: ^(id<MPTransactionProcessTippingAmountStepParametersOptionals> _Nonnull optionals) { [optionals setShowConfirmationScreen:YES]; [optionals setFormatWithIntegerDigits:6 fractionDigits:2]; }]; MPTransactionProcessParameters *pp = [MPTransactionProcessParameters parametersWithSteps: ^(id<MPTransactionProcessParametersSteps> steps) { [steps setTippingStepParameters:params]; }]; UIViewController *viewController = [ui createTransactionViewControllerWithTransactionParameters:tp processParameters:pp completed:/*...*/];
Asking for the Total Amount
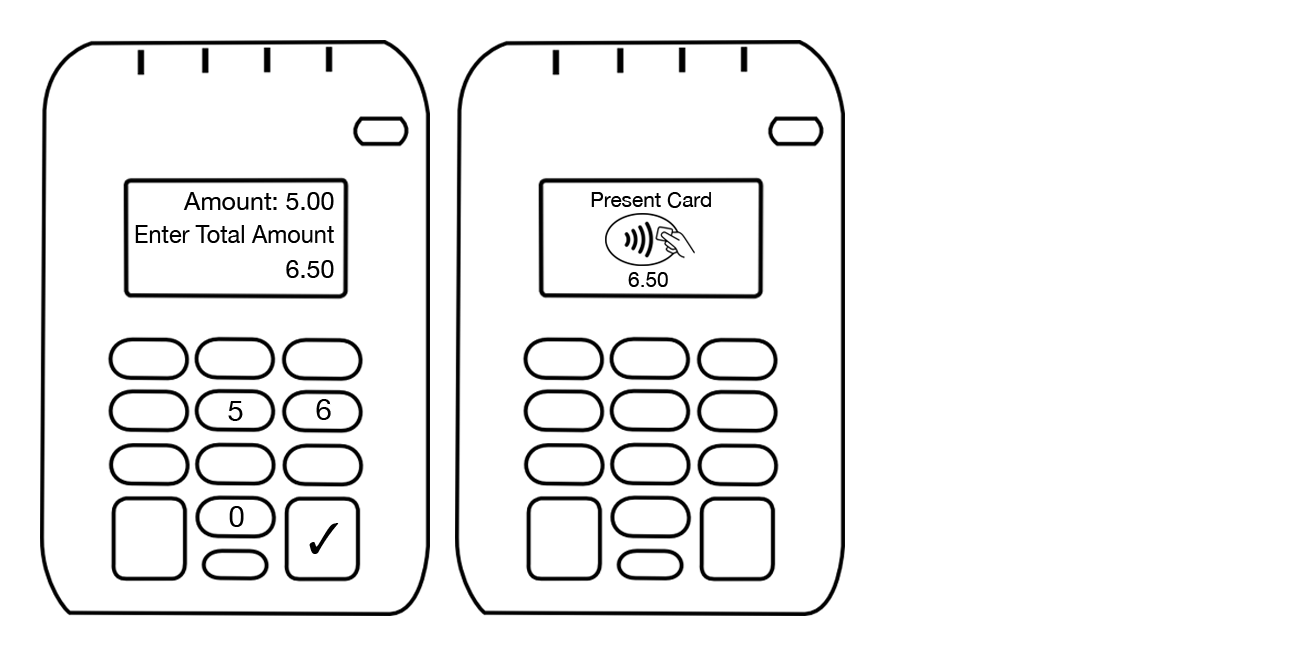
In order to ask the shopper to enter the total amount, including a potential tip, create
MPTransactionProcessTippingStepParameters
with tippingTotaltParametersWithOptionalsBlock
and use them to create the MPTransactionProcessParameters
. Then pass them along as processParameters
to createTransactionViewControllerWithTransactionParameters
:MPTransactionProcessTippingStepParameters *params = [MPTransactionProcessTippingStepParameters tippingTotaltParametersWithOptionalsBlock: ^(id<MPTransactionProcessTippingTotalStepParametersOptionals> _Nonnull optionals) { [optionals setShowConfirmationScreen:NO]; [optionals setFormatWithIntegerDigits:6 fractionDigits:2]; }]; MPTransactionProcessParameters *pp = [MPTransactionProcessParameters parametersWithSteps: ^(id<MPTransactionProcessParametersSteps> steps) { [steps setTippingStepParameters:params]; }]; UIViewController *viewController = [ui createTransactionViewControllerWithTransactionParameters:tp processParameters:pp completed:/*...*/];
Getting the Tip Amount
details.includedTipAmount
property of the MPTransaction
object that you receive as part of the completed
block:
NSLog(@"Included Tip: %@",transaction.details.includedTipAmount);
Asking for a Tip on the Card Reader
Your merchants can easily ask their guest for a tip using On-Reader Tipping. At the start of each transaction, the guest can be asked if they want to give a tip and then can enter the tip amount directly on the card reader. You can also ask the guest to enter the total amount for the transaction, including a potential tip.
On-reader tipping is currently only available on the Miura card readers. To activate this feature, contact your account manager.
Asking for the Tip Amount
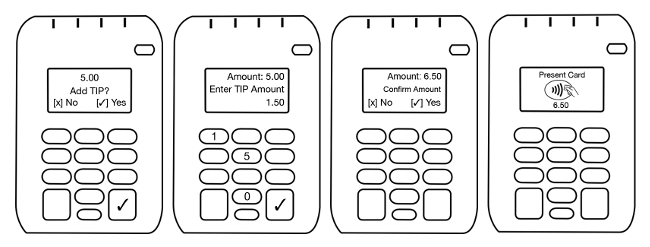
In order to ask the shopper to enter the tip amount, create
TippingProcessStepParameters
with askForTipAmount()
and use them to create the TransactionProcessParameters
. Then, pass along the process parameters to createTransactionIntent
:TippingProcessStepParameters steps = new TippingProcessStepParameters.Builder() .askForTipAmount().showConfirmationScreen(true)//deprecated way to enable add tip screen .showAddTipConfirmationScreen(true) //new way of enabling add tip screen .showTotalAmountConfirmationScreen(true) //enable the total amount confirmation screen .maxTipAmount(new BigDecimal(2.0)) // enables the maximum acceptable tip amount check .numberFormat(6, 2).build(); TransactionProcessParameters transactionProcessParameters = new TransactionProcessParameters.Builder() .addStep(steps) .build(); Intent intent = MposUi.getInitializedInstance().createTransactionIntent(params,transactionProcessParameters); startActivityForResult(intent, MposUi.REQUEST_CODE_PAYMENT);
Asking for the Total Amount
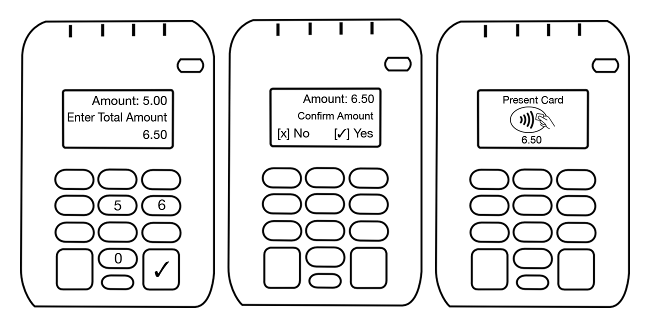
In order to ask the shopper to enter the total amount (including a potential tip), create
TippingProcessStepParameters
with askForTotalAmount()
and use them to create the TransactionProcessParameters
. Then pass along the process parameters to createTransactionInten
:BothTippingProcessStepParameters steps = new TippingProcessStepParameters.Builder() .askForTotalAmount().showConfirmationScreen(false)//deprecated way to disable add tip screen .showAddTipConfirmationScreen(false) //new way of disabling add tip screen .showTotalAmountConfirmationScreen(true) //enable the total amount confirmation screen .maxTipAmount(new BigDecimal(2.0)) // enables the maximum acceptable tip amount check .numberFormat(6, 2).build(); TransactionProcessParameters transactionProcessParameters = new TransactionProcessParameters.Builder() .addStep(steps) .build(); Intent intent = MposUi.getInitializedInstance().createTransactionIntent(params,transactionProcessParameters); startActivityForResult(intent, MposUi.REQUEST_CODE_PAYMENT);
askForTipAmount()
and askForTotalAmount()
tipping strategies benefit from reader screen configuration when performing the transaction; while showAddTipConfirmationScreen()
is used for the first confirmation screen that pops up, showTotalAmountConfirmationScreen()
is managing the second confirmation screen that appears when confirming the total amount of the transaction. The screen configuring properties are optional and by default the add tip configuration screen is enabled and the total amount tip configuration screen is disabled. The maxTipAmount()
is also optional, and when set is defining the upper limit for tip amounts above which shopper will be asked to enter a lesser amount.
For both tipping strategies, the
showConfirmationScreen()
method that configures the first confirmation screen for tipping has been deprecated. Instead, you can use showAddTipConfirmationScreen()
. Getting the Included Tip Amount
getDetails().getIncludedTipAmount()
method of the Transaction
object:
System.out.println("Included Tip: " + transaction.getDetails().getIncludedTipAmount());