Custom Receipts for PAX Terminals
You can print custom receipts for PAX terminals. The Printing API is currently supported on PAX terminals only and is available for Android SDK version 2.64 and up. See more information about printing custom receipts in the Default UI here.
Using the Printing API with TransactionProvider
To use the Printing API with
TransactionProvider
:
- Create a newPrintLayoutusingPrintLayoutFactory.Builder.PrintLayout layout = new PrintLayoutFactory.Builder() .forReceipt(merchantReceipt) .addTransactionInformation() .addMerchantInfo() .build();
- To print a custom receipt, use the new function,TransactionProvider.printCustomReceipt.transactionProvider.printCustomReceipt(layout, accessoryParameters, listener)
Using PrintLayoutFactory.Builder Methods to Configure Custom Receipts
The
PrintLayoutFactory.Builder
includes various methods for configuring custom receipts.- addParagraph()
- This method adds a paragraph with the provided text value. For example, "Thank you for visiting our store today!" (text value). You can specify the alignment and style for the text value.Parameters:
- value:String
- align:Align = LEFT
- textStyle:TextStyle = TextStyle.Default
- addParagraphs()
- This method adds multiple paragraphs from a provided list of text values. For example, "Thank you for visiting our store today!" (text value) and "Check out our newest promotions" (text value). You can specify the alignment and style for the text values.Parameters:
- values:List<String>
- align:Align = LEFT
- textStyle:TextStyle = TextStyle.Default
- addLabelValue()
- This method adds a text label with an associated text value. For example, "Customer ID" (text label) and "01235" (text value). You can specify the style for the text label and value.Parameters:
- label:String
- value:String
- labelStyle:TextStyle = TextStyle.Default
- valueStyle:TextStyle = TextStyle.Default
- addLabelValues()
- This method adds multiple text labels and text values from a provided list of text pairs. For example, "Customer ID" (text label) and "01235" (text value); "Remaining Bonus Points:" (text label) and "987" (text value). You can specify the style for the text labels and values.Parameters:
- values:List<Pair<String, String>>
- labelStyle:TextStyle = TextStyle.Default
- valueStyle:TextStyle = TextStyle.Default
- addImage()
- This method adds an image from the specified URL to the receipt. For example, you could add your merchant's logo to the receipt. The image must be 800px by 800px or less in dimension, and 200KB or less in file size.Parameters:
- url:String
- addEmptyLine()
- This method adds an empty line to the receipt. For example, you could add an empty line to create space between items on the receipt. There are no parameters to configure.
- build()
- This method returns the generatedPrintLayoutobject. There are no parameters to configure.
Using PrintLayoutFactory.Builder Methods with a Customer or Merchant Receipt Object
The
PrintLayoutFactory.Builder
includes these methods for adding detailed information blocks to custom receipts. This process requires passing in a customer or merchant receipt via the forReceipt()
method.- forReceipt()
- This method enables the methods described below to be used with thePrintLayoutFactory.Builder.Parameters:
- receipt:SimpleReceipt. The receipt can be either the merchant or customer receipt object provided by the SDK.
- addTransactionInformation()
- This method adds applicable transaction information to the receipt:
- Transaction type
- Amount and currency
- Subject
- Tipping information
- .addStatusText()
- This method adds the transaction status to the receipt.
- .addPaymentDetails()
- This method adds applicable payment details to the receipt:
- Scheme or label
- Masked account number
- Source
- EMV Application ID
- Account sequence number
- Customer verification
- Remaining balance
- Expiration date
- Magstripe service code
- ATM access code
- Authorization mode
- .addClearingDetails()
- This method adds applicable clearing details to the receipt:
- Transaction identifier
- Original transaction identifier
- Authorization code
- Merchant identifier
- Terminal ID
- Reason code
- .addDccDetails()
- This method adds applicable Dynamic Currency Conversion (DCC) details to the receipt:
- Converted amount and currency
- Conversion rate
- addMerchantInfo()
- This method adds merchant information to the receipt:
- Merchant name
- Merchant address
- addReceiptType()
- This method adds the receipt type to the receipt. Either "Merchant Receipt" or "Customer Receipt" appears on the receipt.
- .addSignatureLines()
- This method adds signature lines to the receipt, if applicable.
- addDateTime()
- This method adds the current time and date to the receipt.
Example Receipt
An example of a receipt that was customized using
PrintLayoutFactory.Builder
methods is shown below.PrintLayout layout = new PrintLayoutFactory.Builder() .forReceipt(merchantReceipt) .addReceiptType() .addMerchantInfo().addEmptyLine() .addTransactionInformation().addEmptyLine().addEmptyLine() .addStatusText().addEmptyLine().addEmptyLine() .addPaymentDetails().addEmptyLine() .addClearingDetails() .addDateTime() .build();
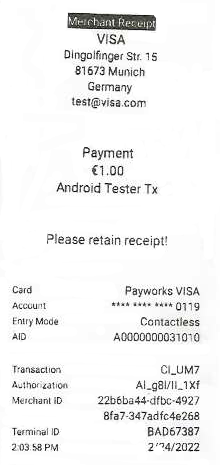