Summary Screen
The Summary screen in the Default UI displays details about the completed transaction on the card reader. This screen displays automatically after every transaction, whether successful or not. You can customize the Summary screen display behavior and features to fit your merchants' needs. A Summary screen example is shown below.
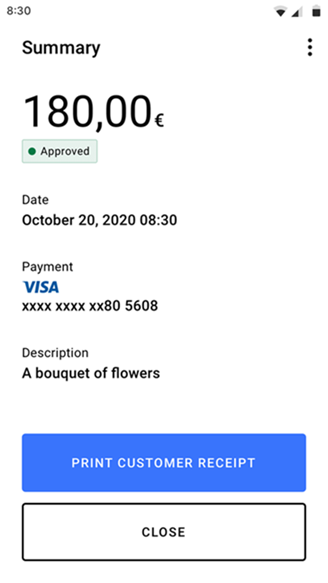
Customizing Display Behavior
You can customize the display behavior of the Summary screen. The display behavior options are shown in the table.
Option | Behavior Description |
---|---|
DISPLAY_INDEFINITELY | Summary screen displays indefinitely. This is the default behavior. |
CLOSE_AFTER_TIMEOUT | Summary screen closes after 5 seconds. |
SKIP_SUMMARY_SCREEN | Summary screen does not display. |
You can change the default display behavior of the Summary screen by setting the
resultDisplayBehavior
property in the UiConfiguration
object.Kotlin
val configuration = UiConfiguration(resultDisplayBehavior = UiConfiguration.ResultDisplayBehavior.CLOSE_AFTER_TIMEOUT) mposUi.configuration = configuration
Java
UiConfiguration configuration = new UiConfiguration.Builder() .resultDisplayBehavior(UiConfiguration.ResultDisplayBehavior.CLOSE_AFTER_TIMEOUT) .build(); mposUi.setConfiguration(configuration);
You can customize the features displayed on the Summary screen. For example, after completing a transaction the merchant might want to refund or capture the transaction, or print a customer receipt. These features can be displayed on the Summary screen. Optional features that can be displayed on the Summary screen are listed in the table and are shown in the Summary screen example below.
Feature | Behavior Description |
---|---|
CAPTURE_TRANSACTION | Capture —Enables the merchant to capture pre-authorized transactions that have not been captured or refunded. |
PRINT_CUSTOMER_RECEIPT | Print customer receipt —Enables the merchant to print the customer receipt. |
PRINT_MERCHANT_RECEIPT | Print merchant receipt —Enables the merchant to print the merchant receipt. |
REFUND_TRANSACTION | Refund —Enables the merchant to process a refund transaction for pre-authorized and captured transactions that have not been refunded. |
SEND_RECEIPT_VIA_EMAIL | Send via email —Enables the merchant to send the customer a receipt through email. |
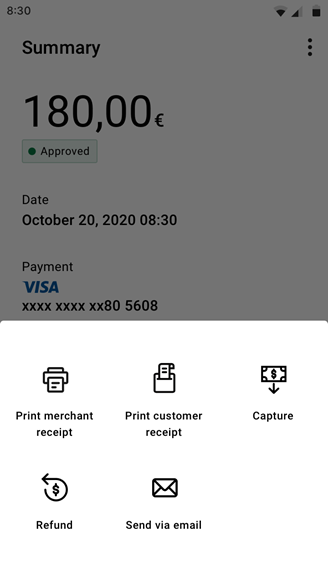
You can change the optional features that display on the Summary screen by setting the
summaryFeatures
property in the UiConfiguration
object.Kotlin
val configuration = UiConfiguration( summaryFeatures = setOf( SummaryFeature.CAPTURE_TRANSACTION, SummaryFeature.PRINT_CUSTOMER_RECEIPT, SummaryFeature.PRINT_MERCHANT_RECEIPT, SummaryFeature.REFUND_TRANSACTION, SummaryFeature.SEND_RECEIPT_VIA_EMAIL ) ) mposUi.configuration = configuration
Java
UiConfiguration configuration = new UiConfiguration.Builder() .summaryFeatures( EnumSet.of( UiConfiguration.SummaryFeature.SEND_RECEIPT_VIA_EMAIL, UiConfiguration.SummaryFeature.PRINT_CUSTOMER_RECEIPT, UiConfiguration.SummaryFeature.PRINT_MERCHANT_RECEIPT, UiConfiguration.SummaryFeature.REFUND_TRANSACTION, UiConfiguration.SummaryFeature.CAPTURE_TRANSACTION ) ) .build(); mposUi.setConfiguration(configuration);
If the summary feature is enabled,
PRINT MERCHANT RECEIPT
will appear as a primary button on the UI by default, as it has a high priority. The default button can also be changed via uiConfiguration
:Kotlin
val configuration = UiConfiguration(defaultSummaryFeature = SummaryFeature.CAPTURE_TRANSACTION) mposUi.configuration = configuration
Java
UiConfiguration configuration = new UiConfiguration.Builder() .defaultSummaryFeature(SummaryFeature.CAPTURE_TRANSACTION) .build(); mposUi.setConfiguration(configuration);
Querying Previous Transactions
If you have the
transactionIdentifier
value of the transaction that you want to query, the Summary screen can display the details for previous transactions. You can access the transactionIdentifier
value in the onActivityResult
callback of the payment method.Kotlin
val summaryIntent = mposUi.createTransactionSummaryIntent(transactionIdentifier = "transactionIdentifier") startActivityForResult(summaryIntent, MposUi.REQUEST_CODE_SHOW_SUMMARY)
Java
Intent summaryIntent = mposUi.createTransactionSummaryIntent("transactionIdentifier"); startActivityForResult(summaryIntent, MposUi.REQUEST_CODE_SHOW_SUMMARY);
You can also override the
onActivityResult
method to retrieve previous transaction information after closing the Summary screen.Kotlin
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { super.onActivityResult(requestCode, resultCode, data) if (requestCode == MposUi.REQUEST_CODE_SHOW_SUMMARY) { when (resultCode) { // Result code from a successful transaction MposUi.RESULT_CODE_APPROVED -> { val transactionIdentifier = data?.getStringExtra(MposUi.RESULT_EXTRA_TRANSACTION_IDENTIFIER) Toast.makeText(findViewById(android.R.id.content),"Transaction approved!\nIdentifier: $transactionIdentifier", Toast.LENGTH_LONG).show() } // Result code from a declined, aborted or failed transaction MposUi.RESULT_CODE_FAILED -> { Toast.makeText(findViewById(android.R.id.content), "Transaction was declined, aborted, or failed", Toast.LENGTH_LONG).show() } } } }
Java
@Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == MposUi.REQUEST_CODE_SHOW_SUMMARY) { if (resultCode == MposUi.RESULT_CODE_APPROVED) { // Transaction was approved String transactionIdentifier = data.getStringExtra(MposUi.RESULT_EXTRA_TRANSACTION_IDENTIFIER); Toast.makeText(this, "Transaction approved, identifier: " + transactionIdentifier, Toast.LENGTH_LONG).show(); } else { // Card was declined, or transaction was aborted, or failed // (e.g. no internet or accessory not found) Toast.makeText(this, "Transaction was declined, aborted, or failed", Toast.LENGTH_LONG).show(); } } }
To query a group of transactions, such as the last 20 transactions performed or transactions that match a specific
customIdentifier
, you can use one of the methods in the transactionModule
:Kotlin
val filter = FilterParameters.Builder() .customIdentifier("my-custom-transaction-id") .build() val includeReceipts = true // Defines if the transaction objects should have receipt information embedded. val offset = 0 // Specifies the offset of the first transaction to return. val limit = 20 //Specifies the maximum number of transactions to return. mposUi.transactionModule.queryTransactions(filter, includeReceipts, offset, limit) { filterParameters, includeReceipts, offset, limit, transactions, error -> run { if (error != null) { // Handle query error } else { // Get the list of matching transactions accessing transactions property } } }
Java
FilterParameters filter = new FilterParameters.Builder() .customIdentifier("my-custom-transaction-id") .build(); Boolean includeReceipts = true; // Defines if the transaction objects should have receipt information embedded. Integer offset = 0; // Specifies the offset of the first transaction to return. Integer limit = 20; //Specifies the maximum number of transactions to return. mposUi.getTransactionModule().queryTransactions(filter, includeReceipts, offset, limit, new QueryTransactionsListener() { @Override public void onCompleted(FilterParameters filterParameters, boolean b, int i, int i1, List<Transaction> list, MposError mposError) { if (mposError != null) { // Handle query error } else { // Get the list of matching transactions accessing list property } } });